technical interview prep
Hey there! If you’re anything like me, you’ve spent much of 2023 preparing for technical interviews.
So, today we’ll do a review of preparation tools that are available to you at varying costs to get yourself ready to go for post-grad technicals (or internship technicals, if you're preparing years in advance).
Then, we’ll touch on two different coding problems that might appear in an interview.
Section 1: Tools
Ok so, right off the bat, let’s dive into tools. All are not created equal, but there are //many!!//
Coding Problem Tools
A large portion of your preparation will be in drilling coding problems. Once upon a time, Cracking the Coding Interview was the only book people used to prepare. Now, there are a lot of different modes of practice out there. Here is a list, with a small description after each one:
LeetCode #You may have heard of LeetCode already, since it’s super popular for skill sharpening among SWEs as much as it is known for providing interview questions. If you haven’t, it’s basically a learning platform, where you can drill coding problems of varying difficulty. Firms pull interview questions directly from here. :) You can also enter contests! Much of the prep content is free.
HackerRank #Super similar to LeetCode. You can prepare for interviews by topic, such as data structures, algs, etc. Again, a lot of free content. Also has competitions!
Daily Coding Problem #Super simple coding problem practice. Made by experts, and solutions aren’t sent until the following morning the problems are released. Free!
Edabit #For fans of Duolingo, the learning interface on Edabit might really be your jive. It’s similar to LeetCode in that there’s coding challenges, but it uses gamification more than any of the others. Free!
CodeSignal #This platform is used by companies to perform remote coding assessments, and they also offer some practice. Some free content, some paid.
Cracking the Coding Interview #Classic book learning written by a former FAANG hiring manager. Amazon $35
CodeAcademy #In addition to lots of introductory courses, it has an interview prep course. $$$
Continuous Learning Tools
3Blue1Brown (3B1B) #3Blue1Brown, also known as Grant Sanderson, is the world’s most popular math teacher. If you ever felt like your linear algebra teacher really flubbed it, Grant’s visuals-first approach to math education has helped literally millions of people feel that “click” sensation. Here are some links for his most popular series: Essence of Linear Algebra; Intro to Neural Networks; Essence of Calculus (Free!)
Khan Academy #Offers solid background on almost any math concept you want, gamified. Free!
Section 2: Parsing Problems
Let’s do some coding problems now. First is a HackerRank easy problem, the next is a LeetCode hard problem.
HackerRank Medium
There is a collection of input strings and a collection of query strings. For each query string, determine how many times it occurs in the list of input strings. Return an array of the results.
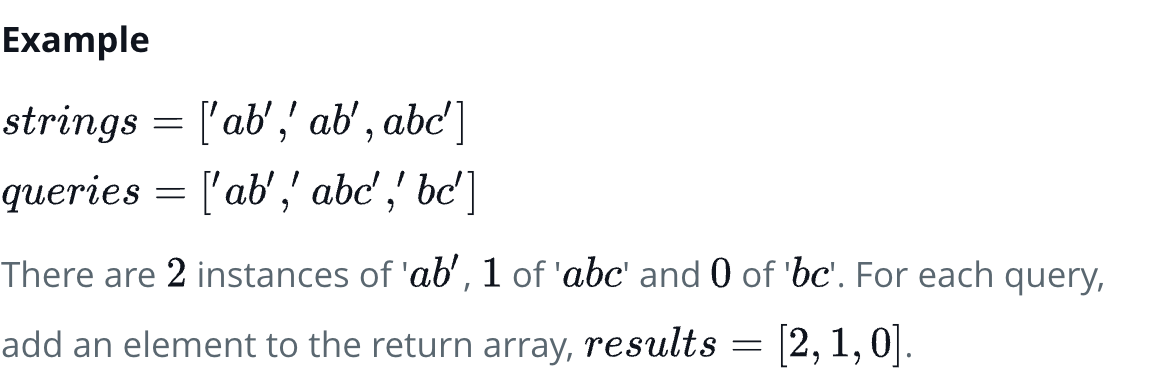
Function Description
Complete the function matchingStrings in the editor below. The function must return an array of integers representing the frequency of occurrence of each query string in strings.
matchingStrings has the following parameters:
strings[n] - an array of strings to search
queries[q] - an array of query strings
Returns
int[q]: an array of results for each query
Input Format
The first line contains and integer n, the size of strings.
Each of the next n lines contains a string strings[i].
The next line contains q, the size of queries.
Each of the next q lines contains a string queries[i].
Constraints
1 < n < 1000
1 < q < 1000
1 < |strings[i]|, |queries[i]| < 20. #All input strings are longer than or equal to 1 char, and all query strings are shorter than or equal to 20 chars
Sample Imput 1
4
aba baba aba xzxb
3
aba xzxb ab
Sample Output 1
2
1
0
Tips: Write some super basic pseudo code before you code anything, or at least have a rough idea of how you will solve. Don’t jump into solving until you’ve worked through any questions you have about problem setup, and your initial thoughts about a solution (in the interview, it’s best to do that out loud! This displays collaborative problem solving skills).
LeetCode Hard
Given two sorted arrays nums1
and nums2
of size m
and n
respectively, return the median of the two sorted arrays.
The overall run time complexity should be O(log (m+n))
.

Constraints:
nums1.length == m
nums2.length == n
0 <= m <= 1000
0 <= n <= 1000
1 <= m + n <= 2000
-106 <= nums1[i], nums2[i] <= 106